Automatically Forward Emails to a Webhook
1. Introduction
Automating email processing is crucial for many applications, from customer support to data integration. With Mockmail's webhook forwarding feature, you can automatically send incoming emails to a webhook URL for real-time processing. This eliminates the need for manual email handling and enables seamless integration with third-party services, such as CRMs, ticketing systems, and notification platforms.
Common Use Cases:
- Automating support ticket creation in helpdesk software.
- Storing customer inquiries in a CRM system.
- Forwarding form submissions to a database or Google Sheets.
- Triggering notifications in Slack or Microsoft Teams.
2. What is a Webhook?
A webhook is a mechanism that allows applications to receive real-time HTTP POST requests whenever a specific event occurs. Unlike traditional polling, where a system repeatedly checks for updates, webhooks push data instantly when an event is triggered. In the context of Mockmail, a webhook allows you to receive incoming emails as structured JSON payloads in real-time, enabling automated workflows and integrations.
3. How Mockmail's Webhook Forwarding Works
When an email is received by your Mockmail inbox, it can be automatically forwarded to a specified webhook URL. The forwarded email includes essential details such as sender address, recipients, email subject & body, and attachments, formatted as JSON.
Key Features:
- Instant email forwarding to any webhook endpoint.
- JSON payload containing email details.
- Support for structured email parsing and attachment handling.
4. Setting Up Webhook Forwarding in Mockmail
Step 1: Create an inbox
First log in to your Mockmail account or create a new one. After sign in click on "New Inbox" on your dashboard page:
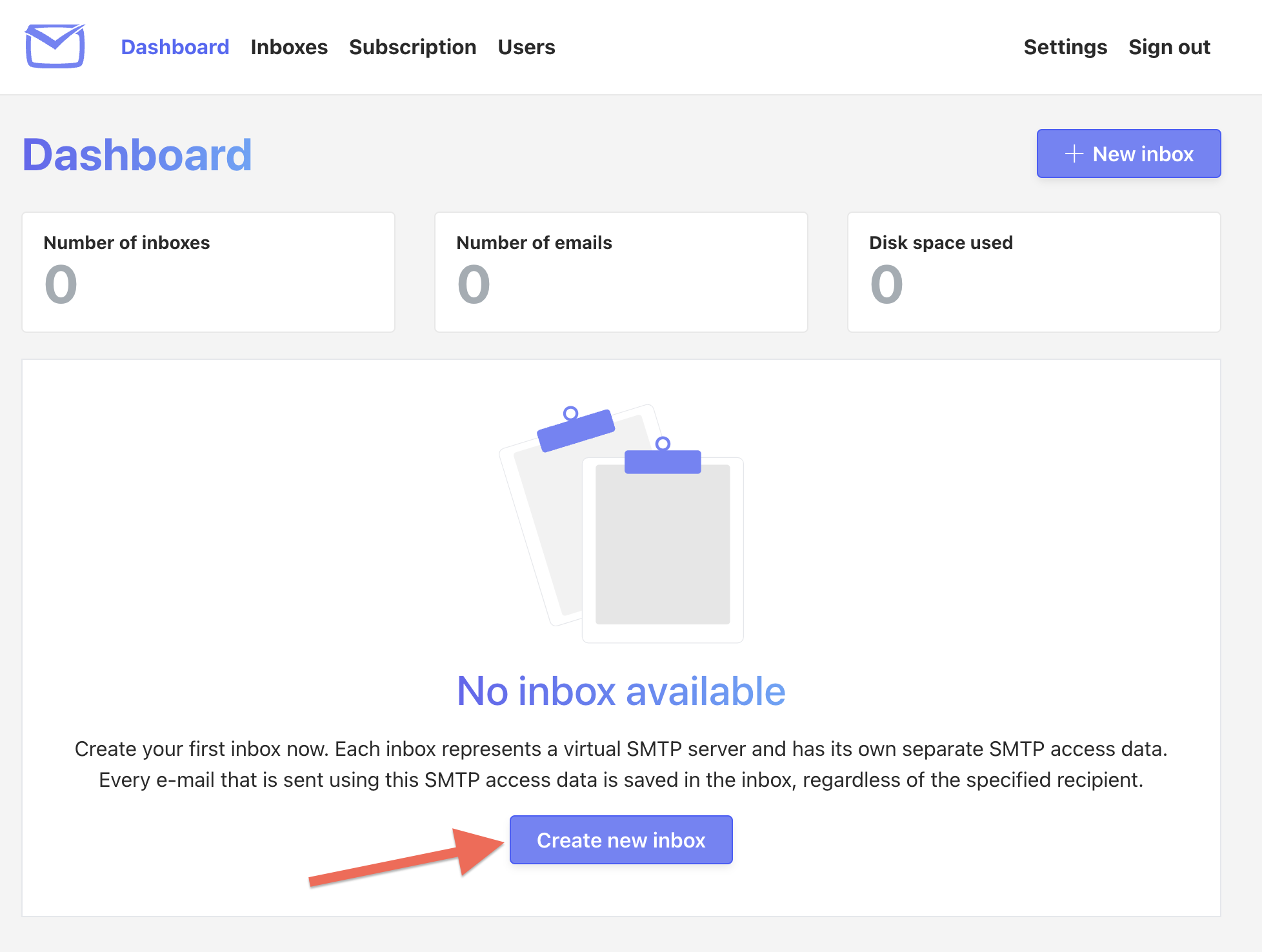
Enter a name for the new Inbox and click on "save" :
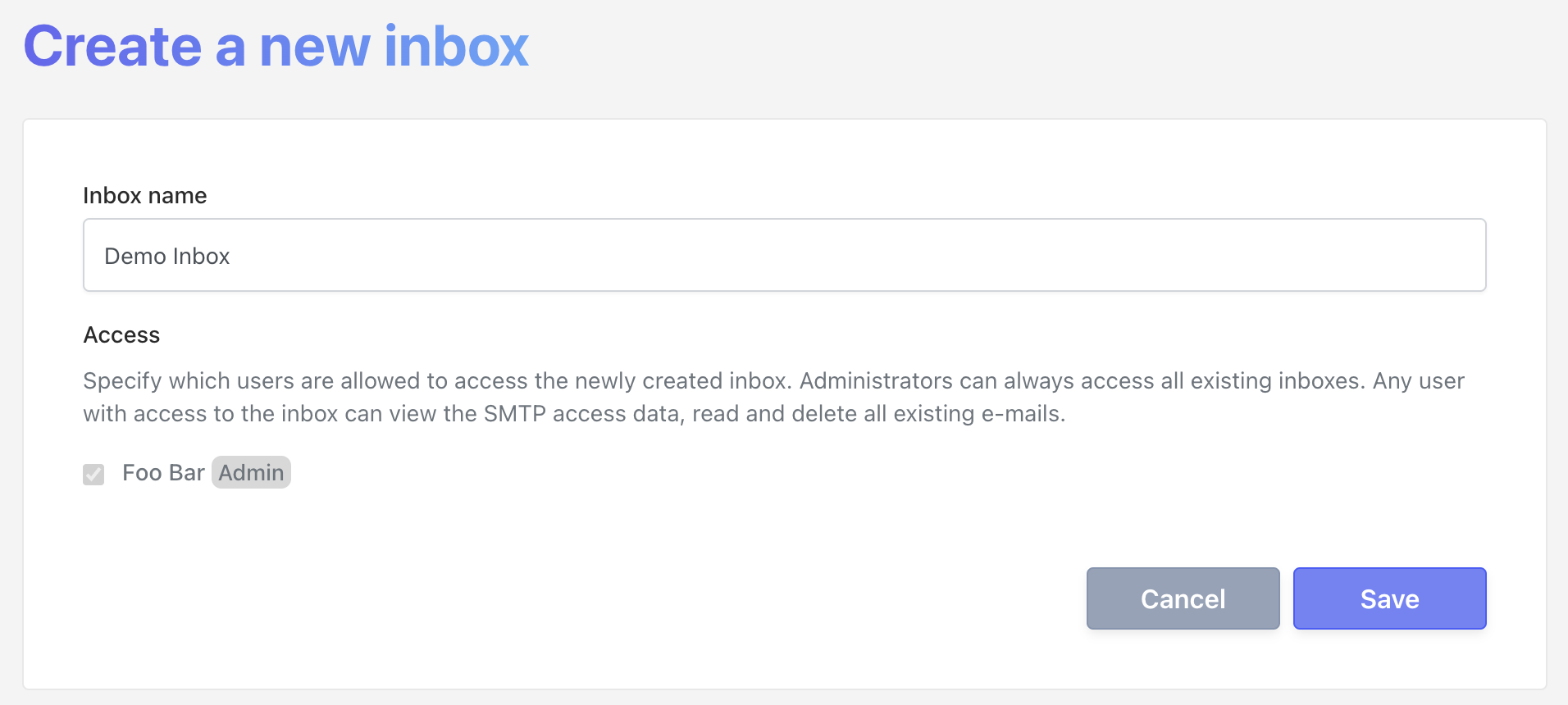
Step 2: Configure the Webhook URL
After inbox creation you are automatically redirected to the newly created inbox. Click on "Webhooks" to get to the webhook settings.
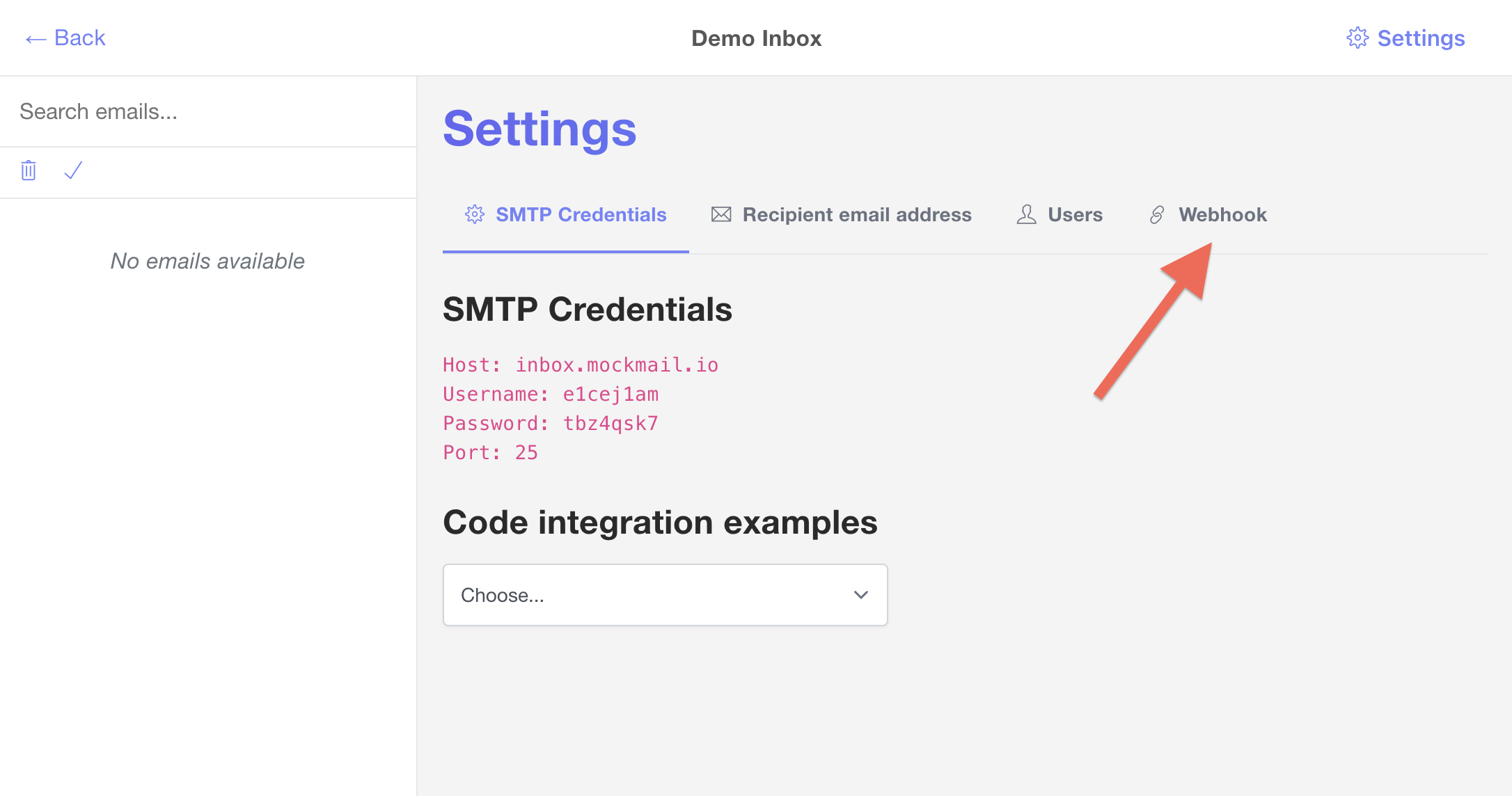
First enable the webhook for the inbox and then enter the URL of your webhook endpoint and click on "Save". To easily test your configuration, click on "Send test event". A demo request will be sent to the webhook URL you have previously defined.
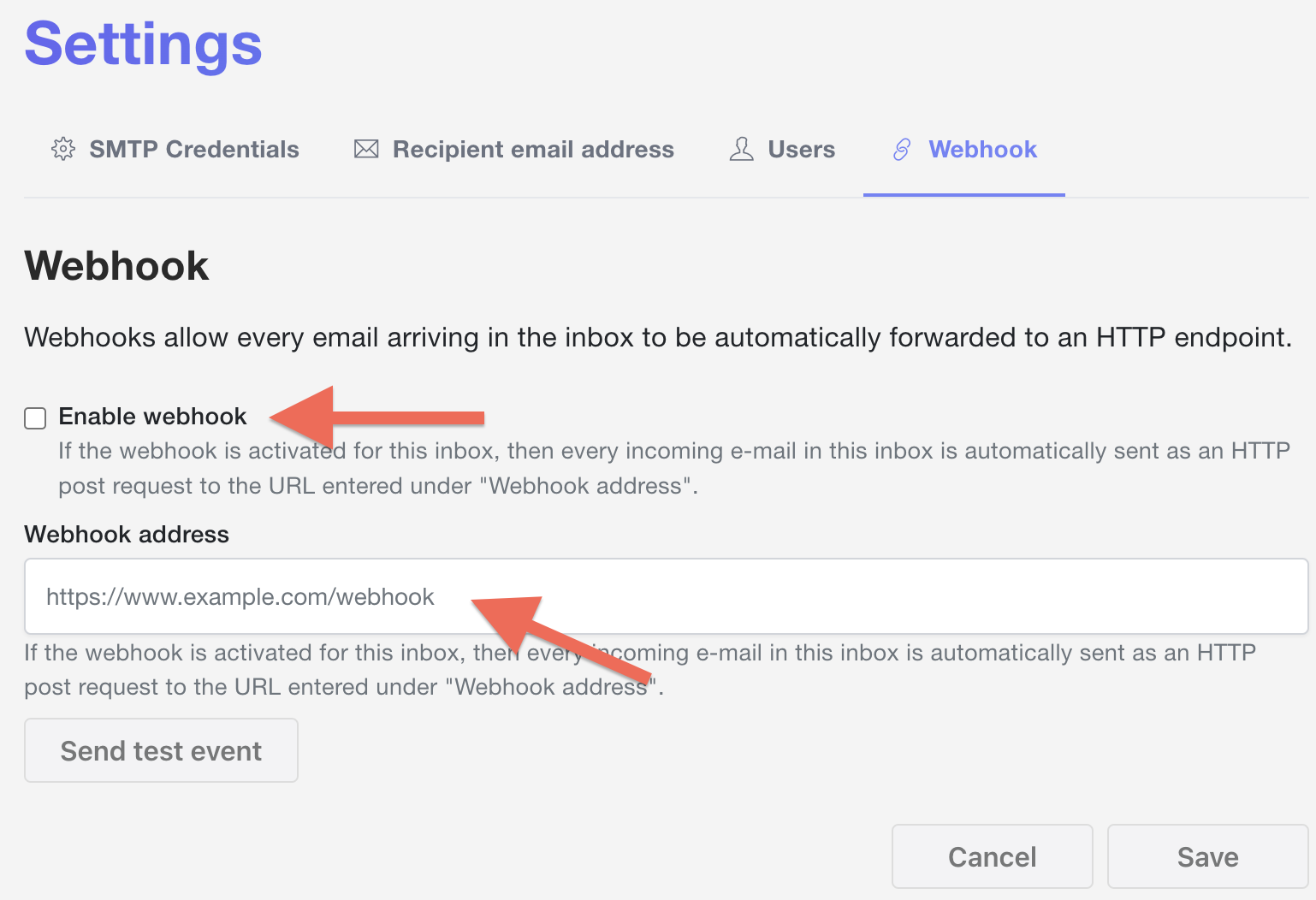
5. Example Webhook Payload
Once an email is received, Mockmail will send a POST request to your webhook URL with a JSON payload similar to the example below:
{
"date": "2025-03-05T12:00:00Z",
"subject": "Sample Webhook E-Mail",
"from_address": "from@example.com",
"from_name": "Mockmail Webhook",
"to_address": "to@example.com",
"content": {
"html": null,
"text": "Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam sit amet tempor orci, quis mollis nisi. Vivamus nec tempor neque. Sed tristique velit eget leo aliquet, nec sollicitudin ipsum consectetur. Integer luctus luctus ex. Mauris molestie diam a cursus dictum. Phasellus feugiat sem vel metus scelerisque auctor."
}
}
6. Handling Incoming Webhook Data in Your Application
Your webhook must be able to receive and process POST requests. Below are simple examples of how to set up a webhook receiver in different programming languages.
Nodejs (Express.js) Example:
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
const PORT = 3000;
app.use(bodyParser.json());
app.post('/webhook', (req, res) => {
const { date, from_address, from_name, to_address, subject, content } = req.body;
console.log(`\n[New Email Received]\nDate: ${date}\nFrom: ${from_name} <${from_address}>\nTo: ${to_address}\nSubject: ${subject}\nContent: ${content.text.substring(0, 50)}...\n`);
res.status(200).json({ message: 'Webhook received successfully' });
});
app.listen(PORT, () => {
console.log(`Mockmail Webhook Server running on http://localhost:${PORT}`);
});
Python (Flask) Example:
from flask import Flask, request
app = Flask(__name__)
@app.route('/webhook', methods=['POST'])
def webhook():
data = request.json
if data:
print("\n[New Email Received]")
print(f"Date: {data.get('date', 'N/A')}")
print(f"From: {data.get('from_name', 'N/A')} <{data.get('from_address', 'N/A')}>")
print(f"To: {data.get('to_address', 'N/A')}")
print(f"Subject: {data.get('subject', 'N/A')}")
print(f"Content: {data.get('content', {}).get('text', 'No content')[:50]}...")
return '', 200
if __name__ == '__main__':
app.run(port=3000, debug=True)